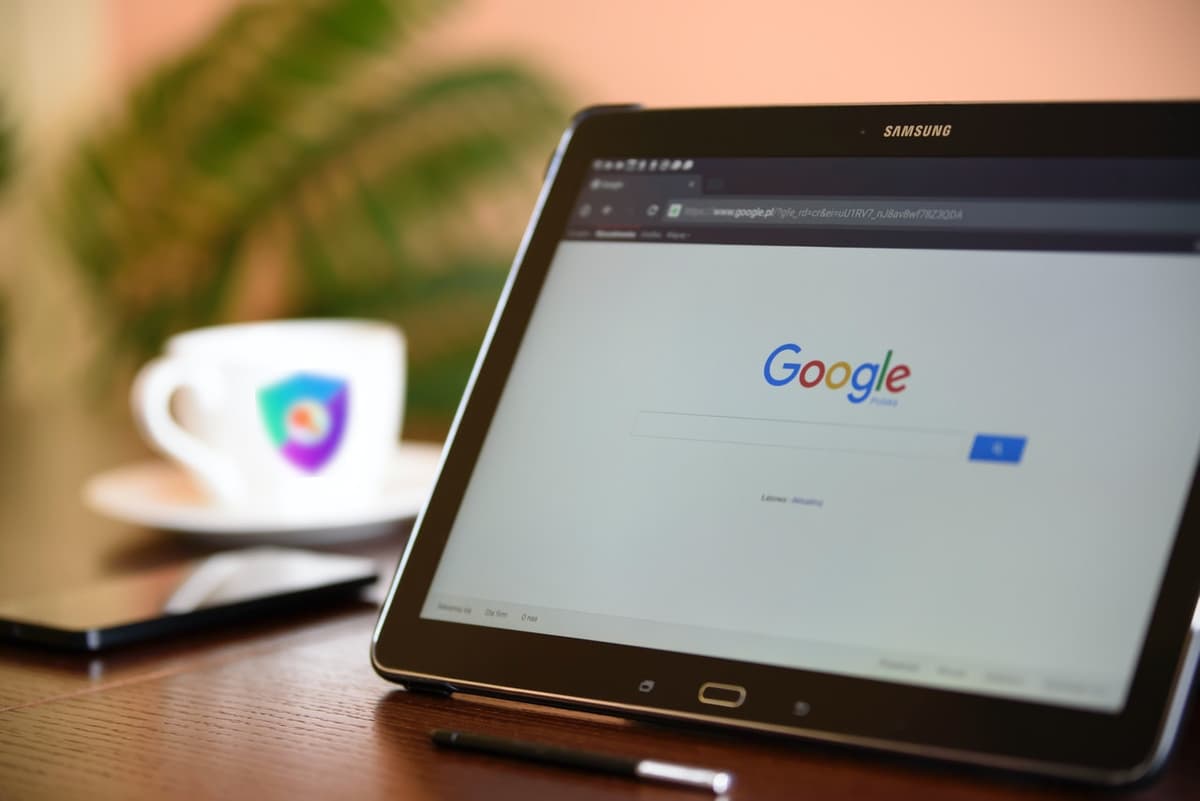
I want to thank Balázs Orbán for helping me writing this tutorial
In this post we're going to explore another way of login into your website powered by NextAuth.js: Google One Tap.
Google One Tap is the quickest way to login through a Google account. Basically the idea is that your website is able to understand if the current user has any Google profile and show a little interface where the user can select which account to use to login, even before starting any login process. So we don't even have to ask the user "Do you want to login with Google?". We already know they can use Google. Here we're going to see how to integrate this login process with NextAuth.js so that we can keep using this library to handle our users and their authentication. I won't go in details about how NextAuth.js works, so I'll assume that you already know about the Credentials Provider and Adapters.
Ingredients and recipe
In order to use Google One Tap, we need to build a custom Credentials Provider
We can configure it to either simply return the user's data, or have access to our user database through an Adapter (or any other way).
Provider
We need to build a custom provider, one that's able to retrieve from Google the information about the user. Once we get the user data, we can return it, or do something else with it, like create the user in our own database.
The Provider is the core part of this login process. We need a Credentials Provider. This provider will receive a token from the front-end generated by Google. We can use this token to query the Google API, retrieve the user and map it to our system. Since we need to interact with Google, we need to get an id and secret from a Google app. You can refer to the Google Provider documentation.
Let's see the code:
Adapter
We don't want to access the database directly, because NextAuth.js already knows how to do so. We can instead use the same adapter we set in NextAuth.js options because that exposes all the needed methods to retrieve or create a user. It's possible to use NexthAuth.js without an adapter: in this case we may decide to implement our own way to access the database but this is definitely less easier. We won't cover this case here.
We want to create an adapter but retain an instance to use it later (in the authorize callback). For this example, we are going to use the MongoDB Adapter, but any other official (or your custom) adapter should do:
Let's see the code:
Front-end
So, on the front-end we need to show the Google One Tap interface (a Google library will do the job) and to pass the token generated by Google to our provider. Usually this interface is shown in the home page, without asking the user to start the login process. This is the power of the Google One Tap, just one click to get in, without ever leaving the homepage of your website.
First of all, let's make sure to include the Google script somewhere in the page. A good place is your _app.tsx and we can use the Script component from Next.js:
We also need to interact with this script. We can create a custom hook to contain the logic
Now, we need to place a div somewhere, with a specific id and use the hook we just created:
That's all.